Splay Trees
Two great things that go great together
Nathan Tenney
WSU Tri-Cities
Splay Trees
- In the discussion of lists, we covered the idea of self-organizing lists.
- A similar concept exists in Binary Search Trees as well.
- As an item is accessed, perform a series of rotations to place the requested node at the root of the tree.
- At worst, operations on a splay tree take
Splay Trees
- Purpose of a splay tree
- Improve access to the most recently sought after item
- Depending on observations, this may include item insertion
- Simple Solution:
- Perform single rotations until the node is at the root
- Improves access to the requested node.
- May make searches for recently accessed items worse
Splay Trees
- Single rotations don’t restructure the tree in a beneficial way
- Instead, consider a series of double rotations to get the node to the root
- Three main cases to consider
- Zig-Zag - Node is a right child of a left child, or a left child of a right child
- Zig-Zig - Node is a right child of a right child, or a left child of a left child
- Zig - Parent is the root
Zig-Zag
- Rotate node around its parent
- Rotate node around its new parent
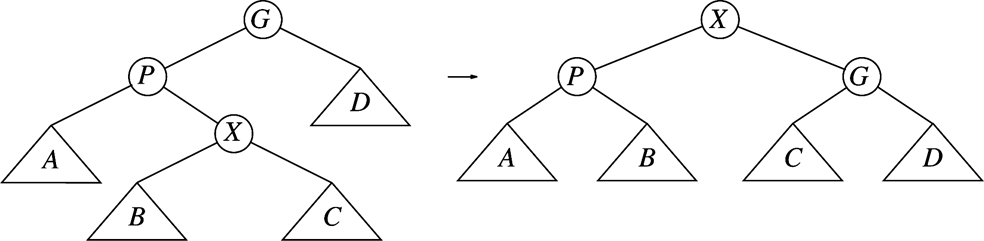
Zig-Zig
- Start at a level above the node.
- Rotate parent around grandparent
- Rotate node around parent
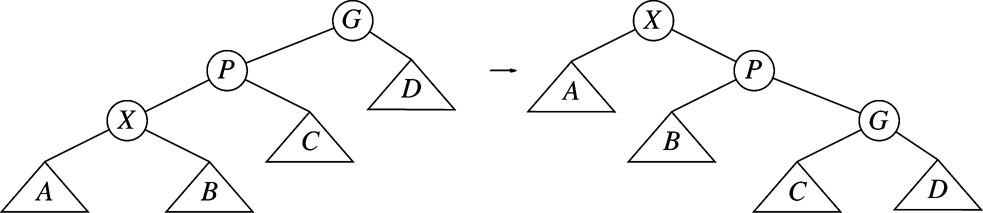
Zig
- Simply rotate node around parent
- Only done as a last step, and only when node is at an odd depth to begin with
Splay Tree Operations
- Operations on a splay tree are done much the same as on other trees
- Insertion and deletion occur at the leaf level
- After each operation, rotations must occur (rotate inserted node to root)
- How is this accomplished?
- Insertion
- Deletion
- Failed Search