Linear Structures
Variations on a Theme
Nathan Tenney
WSU Tri-Cities
Abstract Data Type
- Defined as A set of objects together with a set of operations.
- When we abstract something, we leave out certain details to simplify:
- how the data structure is implemented
- the type of data stored in the data structure
List ADT
- In general, we can consider a list to be a set of objects of the form
- We define a special subset of a list called an empty list which is a list of size 0.
- List of objects may be of any type (most books use integers for simplicity)
- What type of operations might we want to do on a list?
List Implementations
- Two basic implementations of the List ADT:
- Array based
- Linked Structure (Linked List)
The STL Implementations
- STL is an implementation of common data structures
- Uses templates (as discussed previously) to provide an abstract implementation of these container objects
- Big Three functions: size, clear, and empty
- List and vector functions: push_back, pop_back, back, front
- List adds convenience methods for inserting and removing from front
- Vectors add convenience methods including using the [] operator.
Skip List
- Lists have one major drawback
- How can we improve this?
- We add the concept of a Skip List
Skip List
- Provides the ability to perform non-sequential searches on sequential lists.
- Given a skip list of n nodes, for each k and i S.T. and the node in the position points to the node in the position.
- Every node points to the node 2 positions ahead, every node points to the node 4 positions ahead…
- Number of pointers indicates the level of the node
- Max number of levels can be found by
Skip List
- Skip list ideal positioning

Skip List
- What problems, if any, does the skip list have?
- Insertions and deletions may change max level
- Insertions and deletions require some manipulation of the pointers to maintain the structure of the list
- Skip list uneven positioning

- To side step these issues, we randomly select the level of an item being inserted
- In worst case time, this can impact performance, but over the average case, it's close enough to
List Problems
- Searching is the Linked Lists Achilles Heel
- Skip lists were developed to combat this issue
- Skip lists alter the very structure of the list to improve searching. Are there other approaches?
Self-Organizing Lists
- Re-order list items
- New order depends on data
- We will explore 4 methods
- Move-to-Front Method
- Transpose Method
- Count Method
- Ordering Method
Move-To-Front
- Items searched for will be searched for again
- Move the found item to the front of the list
- Guarantees that the most recently found items are near the front of the list
Count Method
- Like the Move-To-Front method, assumes that items searched for will be searched for again
- Nodes in the list keep a count of how many times they've been accessed
- List is ordered by the count
- Ensures that the items most frequently used are near the front of the list
Transpose Method
- Least agressive of the access based methods
- Each time an item is accessed, it is moved forward one spot in the list
- The most used items bubble towards the front of the list.
Insertion of Items
- For each of these, where would it make sense to insert items?
- Move-To-Front
- Count Method
- Transpose Method
Ordering Method
- Data is ordered according to the natural ordering of the elements
- Numeric data
- Alphabetic data
Comparison of Methods
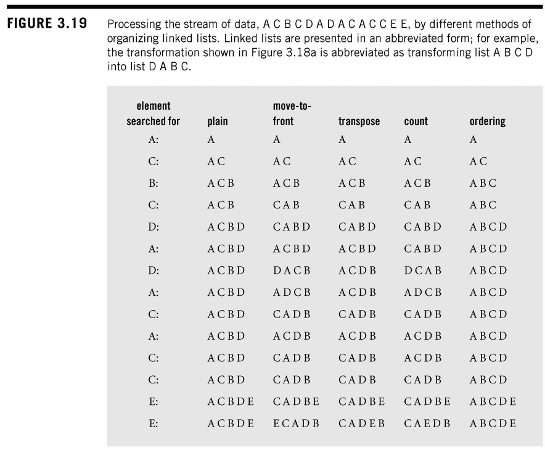
Comparison of Methods
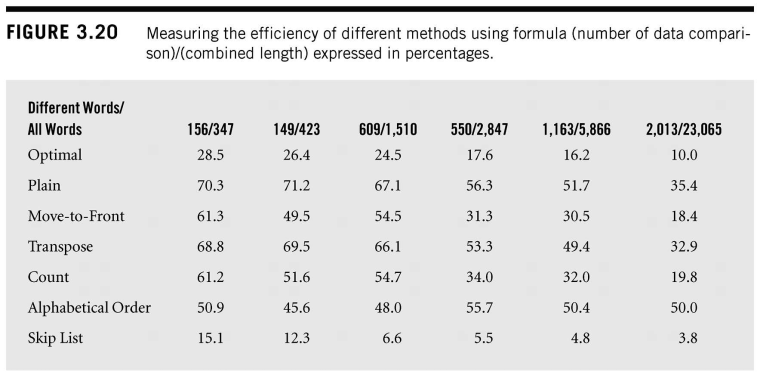
Sparse Tables
- A Table often seems like a good choice to store data
- Consider the case of a university with 8000 students and 300 classes
Sparse Tables
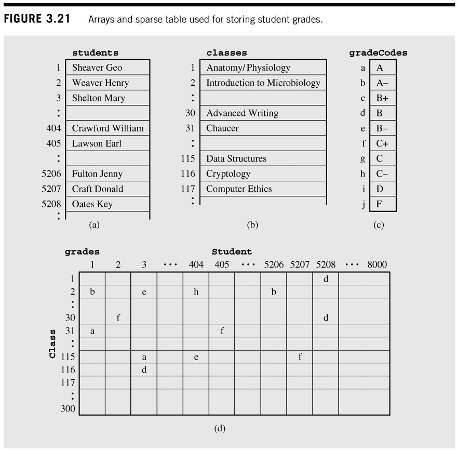
Sparse Tables
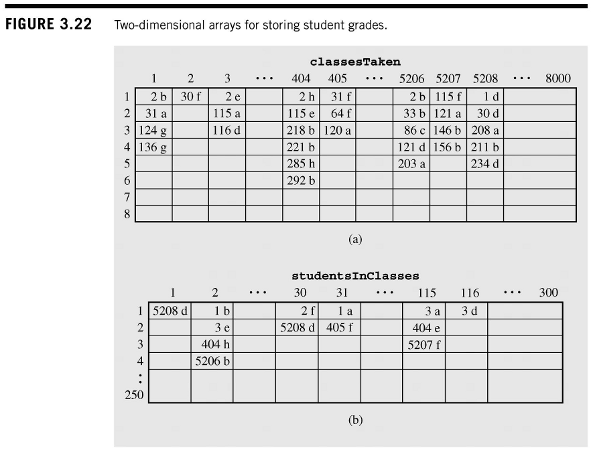
Sparse Tables
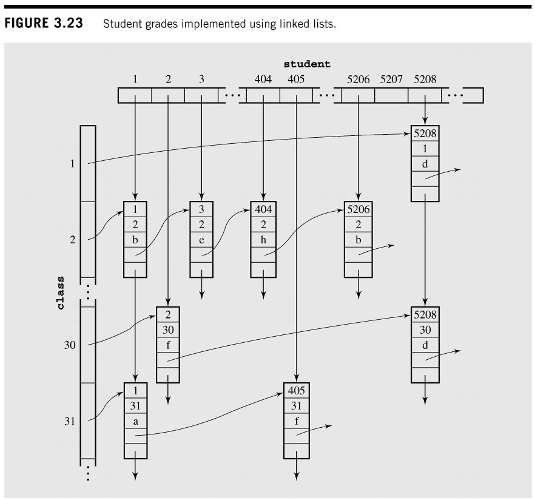
Stacks
- How does a stack differ from a List?
- LIFO
- Methods available in the Stack ADT
- Push - place an element on the stack
- Pop - remove the topmost item from the stack
- Top - examine the topmost element prior to removing it
- Note: pop or top on an empty stack is generally considered an error
- What is the complexity of stack operations?
Stacks
- In general linked lists are the best choice for implementing a stack.
- For special cases, we may need a specific implementation of a stack.
- Linked structure - singly linked list
- Array based - Vector implementation.
Application: Symbol Balancing
- One misplaced symbol can cause pages of errors
- Balance checking program (for simplicity sake we look at balancing parens, brackets and braces)
- The solution turns out to be so simple that most editors/IDE's do this now.
Application: Symbol Balancing
- Each time we encounter an opening symbol, we push that symbol onto a stack
- If the symbol is a closing symbol and the stack is empty, report an error
- If the symbol is a closing symbol, pop a symbol off the stack and compare it to the closing symbol found.
- If they don't match, report an error
- If the stack is not empty at the end of processing, report an error
Application: Postfix Math
Calculating the cost of a shopping trip
-
- 4 function calculator result:
- scientific calculator result:
Application: Postfix Math
- Reason for this is operator precedence
- If we wanted to apply tax only to specific items, first and last for example
-
- Scientific calculator result:
- 4 function calculator result:
Application: Postfix Math
- Essentially, to get the correct result on a normal calculator, we need to keep track of intermediate results
- Alternatively, we can write out the proper order of the sequence of events as follows:
-
- this is known as Postfix Notation or RPN (Reverse Polish Notation)
- easiest way to evaluate is using a stack
- Evaluate the postfix expression:
Application: Postfix Math
Read 6
Application: Postfix Math
Read 5
Application: Postfix Math
Read 2
Application: Postfix Math
Read 3
Application: Postfix Math
Read +
Application: Postfix Math
Read 8
Application: Postfix Math
Read *
Application: Postfix Math
Read +
Application: Postfix Math
Read 3
Application: Postfix Math
Read +
Application: Postfix Math
Read *
|
_ |
|
_ |
|
_ |
Top of stack -> |
288 |
Application: Function Calls
- Two basic things we need to handle when making a function call
- Activation record or stack frame is created an placed on a stack
- As you return from a function call, a stack frame is popped off the stack, and state is restored
- Program continues execution.
- Used in most modern programming languages
Queues
- FIFO - First in First out
- Insertion at the rear of the structure, removal from the front
-
operations, why?
- May use either a list or array implementation
- Circular Array
- Be careful in how you maintain the size of the queue
Queues
- Many different real world situations can be managed by queues
- Hold systems at large businesses
- A whole branch of mathematics, called queueing theory, deals with modeling real world situations utilizing queues
Queues
- Recorded over a period of 3 months
- Number of customers in the bank
- Time it takes to serve them
- Six clerks were employed
- No lines observed
- How to determine the appropriate number of clerks?
Queues
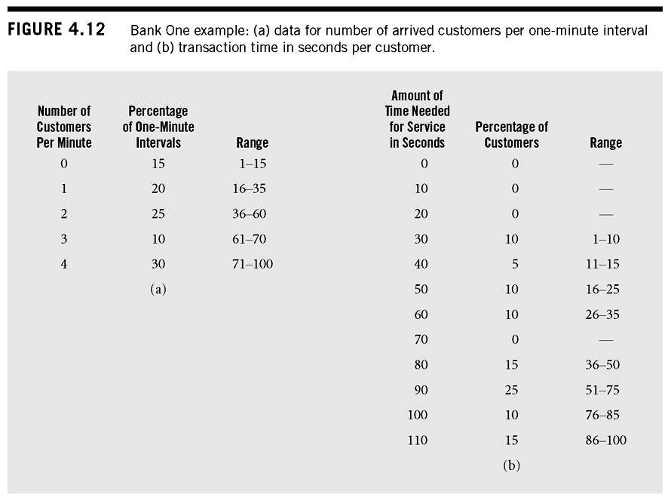
Queues
- Wrote a simulation of customers and tellers
- For each minute
- Number of arriving customers is randomly chosen
- Transaction time for each customer is chosen at arrival time
- As a clerk becomes available, a customer is removed from the queue, and the tellers timer is incremented.
- Run Demo
Priority Queues
- There are instances where a queue is impractical due to priority constraints
- VIP Constraints
- Process scheduling
- Emergency room
- Can be difficult to implement
- Need to find an implementation that keeps enqueue and dequeue operations fast
Priority Queues
- Basic Linked List (2 ways)
- Entry Ordered
- Priority Ordered
- Which is better?
- Two list implementation
- Short, ordered list
- Unordered list
Priority Queues
- Hendriksen's method
- Ordered linked list
- Array of pointers that index into the list
- Comparisons
- Linked list implementations work best on queues of 10 items or less
- Depending on distribution of priorities, 2 list method, comparable to Linked List implementations
- Hendriksen's method performs well on queues of any size.